Builder
The builder pattern is an object creation software design pattern. Unlike the abstract factory pattern and the factory method pattern whose intention is to enable polymorphism, the intention of the builder pattern is to find a solution to the telescoping constructor anti-pattern that occurs when the increase of object constructor parameter combination leads to an exponential list of constructors. Instead of using numerous constructors, the builder pattern uses another object, a builder, that receives each initialization parameter step by step and then returns the resulting constructed object at once.
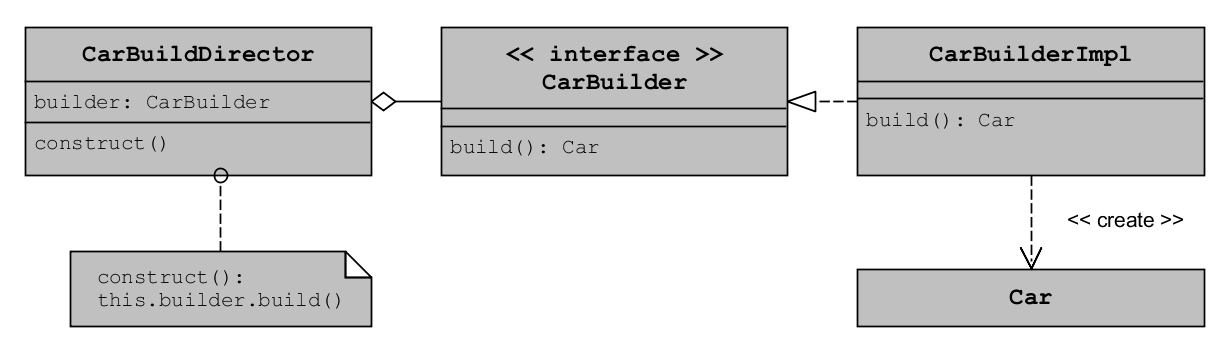
Builder in Java recognizable by creational methods returning the instance itself
java.lang.StringBuilder#append()
(unsynchronized)java.lang.StringBuffer#append()
(synchronized)java.nio.ByteBuffer#put()
(also onCharBuffer
,ShortBuffer
,IntBuffer
,LongBuffer
,FloatBuffer
andDoubleBuffer
)javax.swing.GroupLayout.Group#addComponent()
- All implementations of
java.lang.Appendable
/**
* Represents the product created by the builder.
*/
class Car {
private int wheels;
private String color;
public Car() {
}
public String getColor() {
return color;
}
public void setColor(final String color) {
this.color = color;
}
public int getWheels() {
return wheels;
}
public void setWheels(final int wheels) {
this.wheels = wheels;
}
@Override
public String toString() {
return "Car [wheels = " + wheels + ", color = " + color + "]";
}
}
/**
* The builder abstraction.
*/
interface CarBuilder {
Car build();
CarBuilder setColor(final String color);
CarBuilder setWheels(final int wheels);
}
class CarBuilderImpl implements CarBuilder {
private Car car;
public CarBuilderImpl() {
car = new Car();
}
@Override
public Car build() {
return car;
}
@Override
public CarBuilder setColor(final String color) {
car.setColor(color);
return this;
}
@Override
public CarBuilder setWheels(final int wheels) {
car.setWheels(wheels);
return this;
}
}
public class CarBuildDirector {
private CarBuilder builder;
public CarBuildDirector(final CarBuilder builder) {
this.builder = builder;
}
public Car construct() {
return builder.setWheels(4)
.setColor("Red")
.build();
}
public static void main(final String[] arguments) {
final CarBuilder builder = new CarBuilderImpl();
final CarBuildDirector carBuildDirector = new CarBuildDirector(builder);
System.out.println(carBuildDirector.construct());
}
}