Chain of Responsibility
In object-oriented design, the chain-of-responsibility pattern is a design pattern consisting of a source of command objects and a series of processing objects. Each processing object contains logic that defines the types of command objects that it can handle; the rest are passed to the next processing object in the chain. A mechanism also exists for adding new processing objects to the end of this chain.
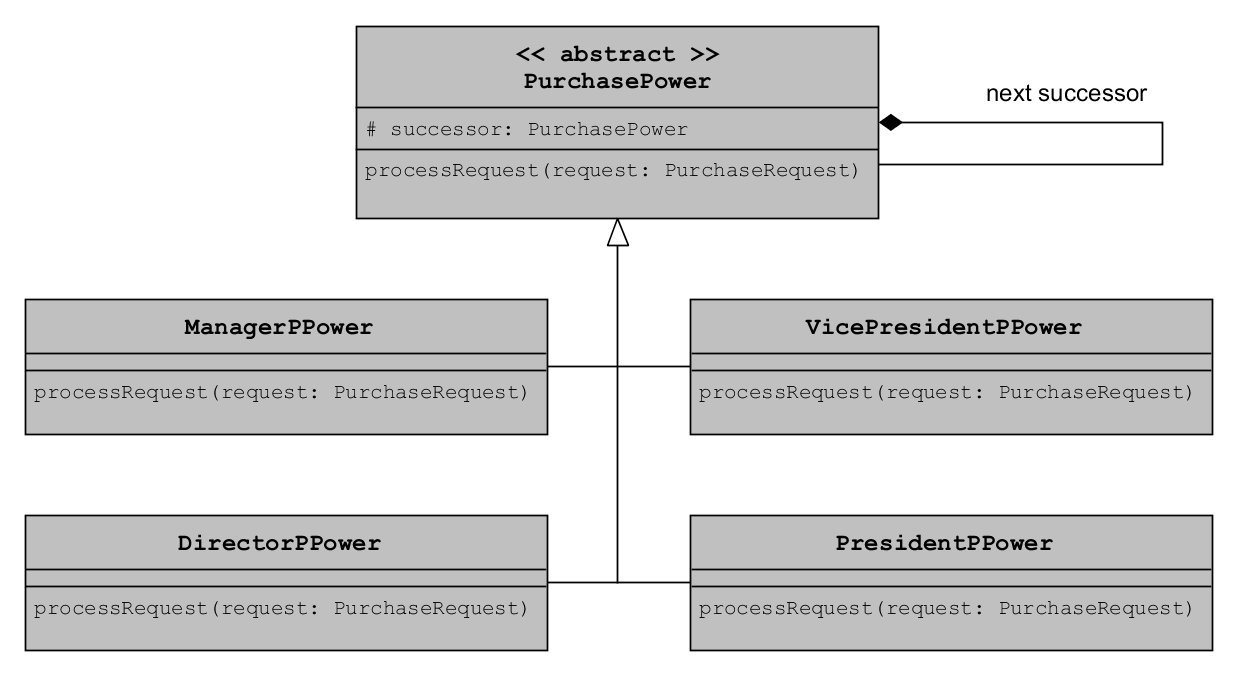
Chain of Responsibility in Java recognizable by behavioral methods which (indirectly) invokes the same method in another implementation of same abstract/interface type in a queue)
java.util.logging.Logger#log()
javax.servlet.Filter#doFilter()
abstract class PurchasePower {
protected static final double BASE = 500;
protected PurchasePower successor;
abstract protected double getAllowable();
abstract protected String getRole();
public PurchasePower(PurchasePower successor) {
this.successor = successor;
}
public void setSuccessor(PurchasePower successor) {
this.successor = successor;
}
public void processRequest(PurchaseRequest request) {
if (request.getAmount() < this.getAllowable()) {
System.out.println(this.getRole() +
" will approve $" + request.getAmount());
} else if (successor != null) {
successor.processRequest(request);
}
}
}
class ManagerPPower extends PurchasePower {
protected double getAllowable() {
return BASE * 10;
}
protected String getRole() {
return "Manager";
}
}
class DirectorPPower extends PurchasePower {
protected double getAllowable() {
return BASE * 20;
}
protected String getRole() {
return "Director";
}
}
class VicePresidentPPower extends PurchasePower {
protected double getAllowable() {
return BASE * 40;
}
protected String getRole() {
return "Vice President";
}
}
class PresidentPPower extends PurchasePower {
protected double getAllowable() {
return BASE * 60;
}
protected String getRole() {
return "President";
}
}
class PurchaseRequest {
private double amount;
private String purpose;
public PurchaseRequest(double amount, String purpose) {
this.amount = amount;
this.purpose = purpose;
}
public double getAmount() {
return this.amount;
}
public void setAmount(double amount) {
this.amount = amount;
}
public String getPurpose() {
return this.purpose;
}
public void setPurpose(String purpose) {
this.purpose = purpose;
}
}
class CheckAuthority {
public static void main(String[] args) {
PresidentPPower president = new PresidentPPower();
VicePresidentPPower vp = new VicePresidentPPower();
vp.setSuccessor(president);
DirectorPPower director = new DirectorPPower();
director.setSuccessor(vp);
ManagerPPower manager = new ManagerPPower();
manager.setSuccessor(director);
try {
while (true) {
System.out.println("Enter the amount to check who " +
"should approve your expenditure.");
System.out.print(">");
double d = Double.parseDouble(new BufferedReader(
new InputStreamReader(System.in)).readLine());
manager.processRequest(new PurchaseRequest(d, "General"));
}
}
catch (Exception exc) { System.exit(1); }
}
}