Unified Modeling Language
- Access Level
+
field or method with public access level-
field or method with private access level#
field or method with protected access level~
field or method with no modifier or tilde has package access level- Attributes
static
fields or methods are underlined,STATIC
fields are uppercase<< final >>
field attribute can be added using guillemets (<< >>
) (not required)- Fields & Methods
fieldName: Type
field with fieldName and return of TypemethodName(param: ParamType): Type
method with methodName, param of ParamType and return of Type- Abstraction/Initialization
abstract
or<< abstract >>
classes are italicized or use guillemets<< interface >>
use guillemets<< constructor >>
use guillemets- Relationships
- unidirectional
A ——> B
, A has a dependency on B (A has a field of type B), A is aware of the relationship, B is not - bidirectional
A ——— B
orA <——> B
, both classes are aware of (have access to) each other - Relationship Multiplicity
0..1
- Zero or One1
- Only One*
- Zero or More0..*
- Zero or More1..*
- One or More2
- Two Only0..3
- Zero to Three2..5
- Two to Five
UML Relationships
Dependency
Controller depends on UserService, very loose, decoupled relationship. Dependency class UserService is injected, imported or method created. Class UserController does not have a field of type UserService.

Controller depends on UserService, very loose, decoupled relationship. Dependency class UserService is injected, imported or method created. Class UserController does not have a field of type UserService.
import UserService; // dependency imported
@Inject UserService userService; // dependency injected
public class UserController() {
public Integer getAge() {
// calc age using userService
{
// dependency method creation
public UserService getUserService() {
return new UserService();
}
}
Association
Association dependency indicates Canvas has a field of type Shape. Canvas is aware of Shape, but Shape is not aware of Canvas. (Arrow indicates a one-way relationship.)

Association dependency indicates Canvas has a field of type Shape. Canvas is aware of Shape, but Shape is not aware of Canvas. (Arrow indicates a one-way relationship.)
public class Canvas() {
private Shape square;
public Canvas(Square square) {
this.square = square;
{
public void draw() {
this.square.draw();
}
}
Aggregation
Car and Storage contain a reference to an instance of Wheel as part of Car's and Storage's state. The end of the aggregator

Car and Storage contain a reference to an instance of Wheel as part of Car's and Storage's state. The end of the aggregator
Car, Storage
lifetime may or may-not destroy the aggregated Wheel
instances.
public class Wheel {
private int size;
}
public class Car {
private List<Wheel> list = new ArrayList<>(4);
// mutator methods
}
public class Storage {
private List<Wheel> list = new ArrayList<>();
// mutator methods
}
Composition
Similar to aggregation except child class instance lifecycle is dependent on the parent class instance lifecycle. Destruction of parent container destroys it's children.

Similar to aggregation except child class instance lifecycle is dependent on the parent class instance lifecycle. Destruction of parent container destroys it's children.
public class Company {
private List<Department> departments = new ArrayList<>();
public Company() {
department.add(new Department("Sales"));
department.add(new Department("R&D"));
}
// Company does not export Department
}
Generalization
Symbolizes general class inheritance, in this instance the SubClass will inherit from SuperClass.

Symbolizes general class inheritance, in this instance the SubClass will inherit from SuperClass.
public class SubClass extends SuperClass {}
Realization
Interfaces allow the implementation of specific behavior.

Interfaces allow the implementation of specific behavior.
public class Eye interface Comparable<Eye> {
public int compareTo(Eye eye) {}
}
Class Example w/ Inheritance & Abstraction
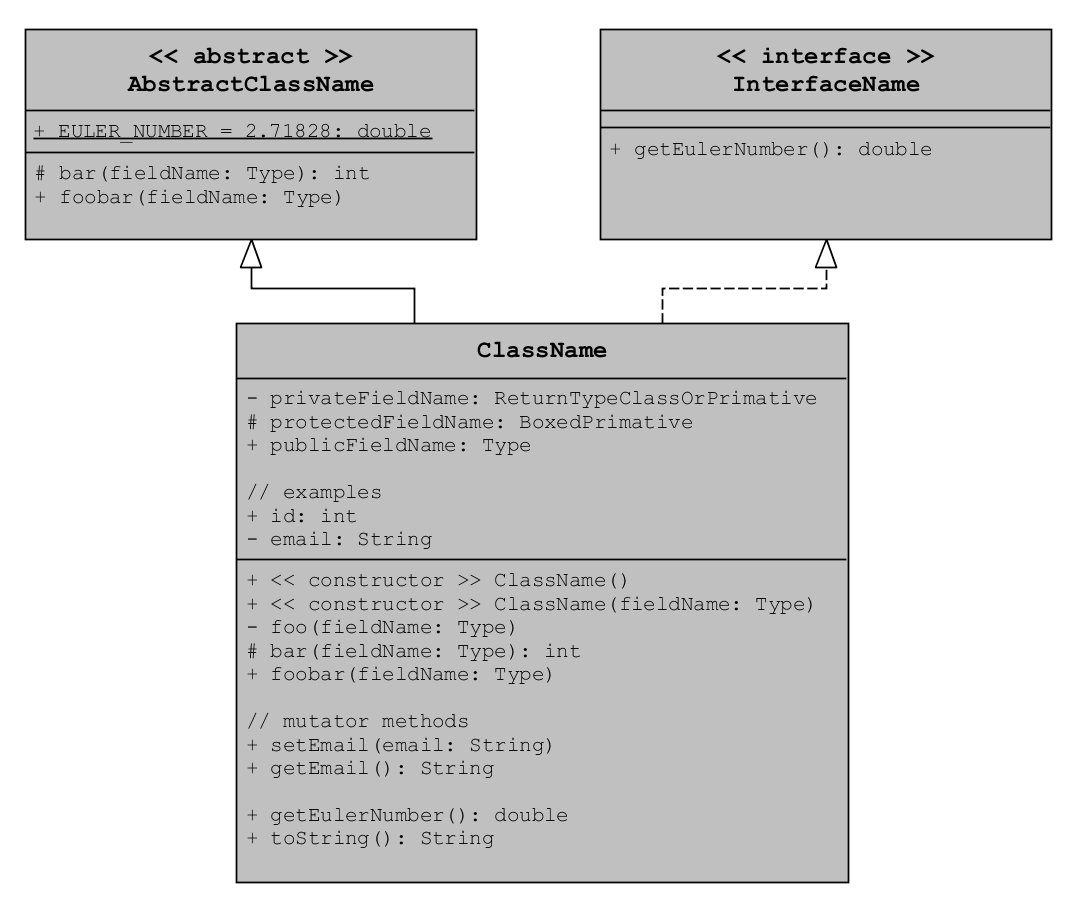
public interface InterfaceName {
public double getEulerNumber();
}
public abstract class AbstractClassName {
public static final double EULER_NUMBER = 2.71828d;
protected abstract int bar(Type fieldName);
public abstract void foobar(Type fieldName);
}
public class ClassName extends AbstractClassName implements InterfaceName {
private ReturnTypeClassOrPrimative privateFieldName;
protected BoxedPrimative protectedFieldName;
public Type publicFieldName;
// examples
public int id;
private String email;
public ClassName() {
// init code
}
public ClassName(Type fieldName) {
// init code
}
private void foo(Type fieldName) {
// method code
}
@Override
protected int bar(Type fieldName) {
// method code
return 0;
}
@Override
public void foobar(Type fieldName) {
// method code
}
// mutator methods
public void setEmail(String email) {
this.email = email;
}
public String getEmail() {
return email;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder();
// to string code
return sb.toString();
}
@Override
public double getEulerNumber() {
return EULER_NUMBER;
}
}