Business Delegate
Business Delegate Pattern is used to decouple business tier and presentation tier. It is use to reduce communication or remote lookup functionality to business tier code in presentation tier code.
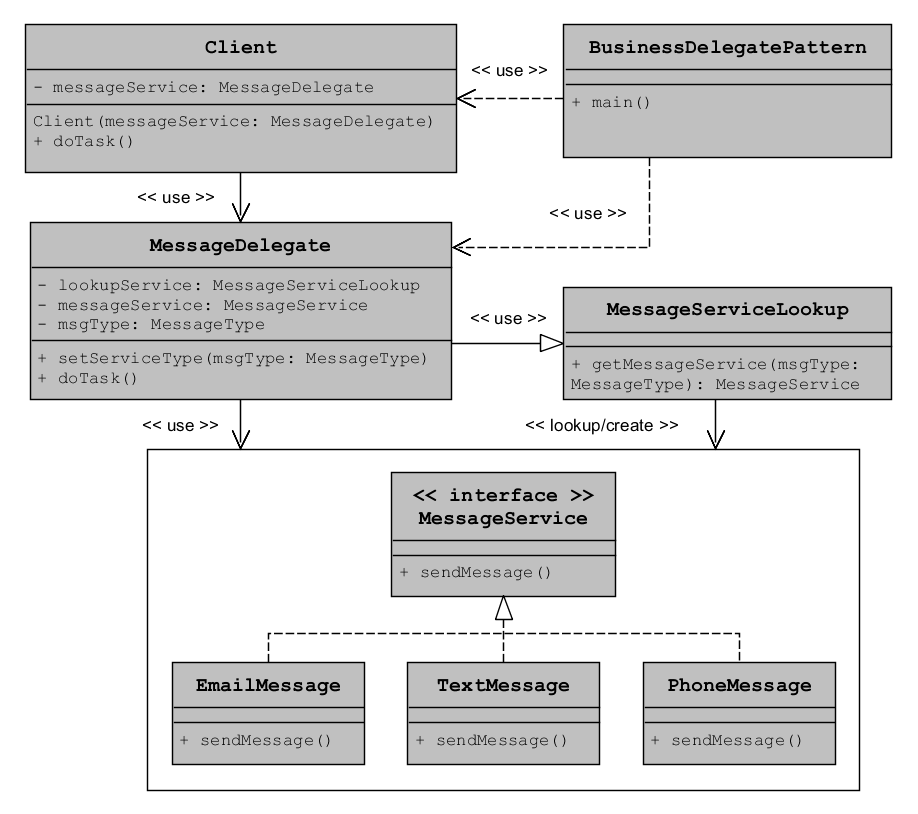
public interface MessageService {
public void sendMessage();
}
public class EmailMessage implements MessageService {
@Override
public void sendMessage() {
System.out.println("Send Email");
}
}
public class TextMessage implements MessageService {
@Override
public void sendMessage() {
System.out.println("Send Text Message");
}
}
public class PhoneMessage implements MessageService {
@Override
public void sendMessage() {
System.out.println("Phone Call");
}
}
public class MessageServiceLookup {
public MessageService getMessageService(MessageType msgType) {
switch(msgType) {
case EMAIL: return new EmailMessage();
case TEXT: return new TextMessage();
default: return new PhoneMessage();
}
}
}
public class MessageDelegate {
private final MessageServiceLookup lookupService = new MessageServiceLookup();
private MessageService messageService;
private MessageType msgType;
public void setServiceType(MessageType msgType) {
this.msgType = msgType;
}
public void doTask() {
messageService = lookupService.getMessageService(msgType);
messageService.sendMessage();
}
}
public class Client {
private final MessageDelegate messageService;
public Client(MessageDelegate messageService) {
this.messageService = messageService;
}
public void doTask() {
messageService.doTask();
}
}
public class BusinessDelegatePattern {
public static void main(String[] args) {
MessageDelegate messageDelegate = new MessageDelegate();
messageDelegate.setServiceType(MessageType.EMAIL);
Client client = new Client(messageDelegate);
client.doTask();
messageDelegate.setServiceType(MessageType.TEXT);
client.doTask();
}
}